Principle Of RFID:
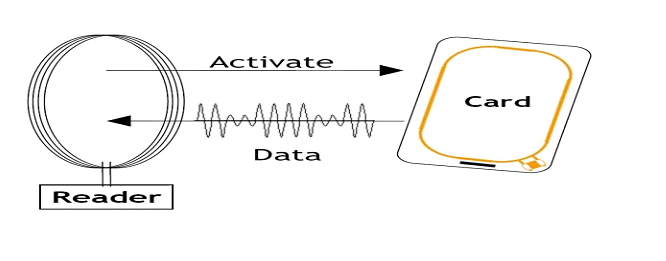
RFID READER MODULE

Pin Description

INTCON (Interrupt Control Register)

PEIE/GIEL:
GIE/GIEH:
PIR1 (Peripheral Interrupt Request 1)

TXIF
RCIF
TXREG
PIE1 (Peripheral Interrupt Enable 1)

TXIE
RCIE
Applications
/* Name : main.c * Purpose : Source code for RFID interface with ATMEGA16. * Author : Gemicates * Date : 2017-09-11 * Website : www.gemicates.org * Revision : None */ // Program to get the 12 byte string and display it on LCD by Polling method: /* The RFID unique code is been displayed on LCE LCD DATA port----PORT B ctrl port------PORT D rs-------PD0 rw-------PD1 en-------PD2 */ #define F_CPU 8000000UL #define USART_BAUDRATE 9600 #define BAUD_PRESCALE (((F_CPU / (USART_BAUDRATE * 16UL))) - 1) #include<avr/io.h> #include<util/delay.h> #define delay_ms; #define LCD_DATA PORTA // LCD data port #define ctrl PORTB #define en PB2 // enable signal #define rw PB1 // read/write signal #define rs PB0 // register select signal void LCD_cmd(unsigned char cmd); void init_LCD(void); void LCD_write(unsigned char data); void usart_init(); unsigned int usart_getch(); unsigned char i, card[12]; void getcard_id(void); void LCD_display(void); int main(void) { DDRA=0xff; // LCD_DATA port as output port DDRB=0x07; // ctrl as output init_LCD(); // initialization of LCD delay_ms(50); // delay of 50 milliseconds usart_init(); // initiailztion of USART LCD_write_string("Unique ID No."); // Function to display string on LCD while(1) { getcard_id(); // Function to get RFID card no. from serial port LCD_cmd(0xC0); // to go in second line and zeroth position on LCD LCD_display(); // a function to write RFID card no. on LCD } return 0; } void getcard_id(void) // Function to get 12 byte ID no. from rfid card { for(i=0;i<12;i++) { card[i]= usart_getch(); // receive card value byte by byte } return; } void LCD_display(void) // Function for displaying ID no. on LCD { for(i=0;i<12;i++) { LCD_write(card[i]); // display card value byte by byte } return; } void init_LCD(void) { LCD_cmd(0x38); // initializtion of 16x2 LCD in 8bit mode _delay_ms(1); LCD_cmd(0x01); // clear LCD _delay_ms(1); LCD_cmd(0x0E); // cursor ON _delay_ms(1); LCD_cmd(0x80); // ---8 go to first line and --0 is for 0th position _delay_ms(1); return; } void LCD_cmd(unsigned char cmd) { LCD_DATA=cmd; ctrl =(0<<rs)|(0<<rw)|(1<<en); _delay_ms(1); ctrl =(0<<rs)|(0<<rw)|(0<<en); _delay_ms(50); return; } void LCD_write(unsigned char data) { LCD_DATA= data; ctrl = (1<<rs)|(0<<rw)|(1<<en); _delay_ms(1); ctrl = (1<<rs)|(0<<rw)|(0<<en); _delay_ms(50); return ; } void usart_init() { UCSRB |= (1 << RXEN) | (1 << TXEN); // Turn on the transmission and reception circuitry UCSRC |= (1 << URSEL) | (1<<USBS) | (1 << UCSZ0) | (1 << UCSZ1); // Use 8-bit character sizes UBRRL = 0x51; // Load lower 8-bits of the baud rate value.. // into the low byte of the UBRR register UBRRH = 0x103; // Load upper 8-bits of the baud rate value.. // into the high byte of the UBRR register } unsigned int usart_getch() { while ((UCSRA & (1 << RXC)) == 0); // Do nothing until data have been received.. // and is ready to be read from UDR return(UDR); // return the byte } void LCD_write_string(unsigned char *str) // take address value of the string in pointer *str { int i=0; while(str[i]!='\0') // loop will go on till the NULL characters is soon in string { LCD_write(str[i]); // sending data on LCD byte by byte i++; } return; }